SDK Quickstart
The fastest way to connect your dApp to the blockchain and get alerts with block time streamed data.
Table of Contents
Getting Started
Check out the npm package here:
1. Get Your API Key (bearer token)
To access Hello Moon's free data, you need an API key to authenticate your requests and messages. You can create or find your API Key from our dashboard. Visit https://www.hellomoon.io/dashboard.
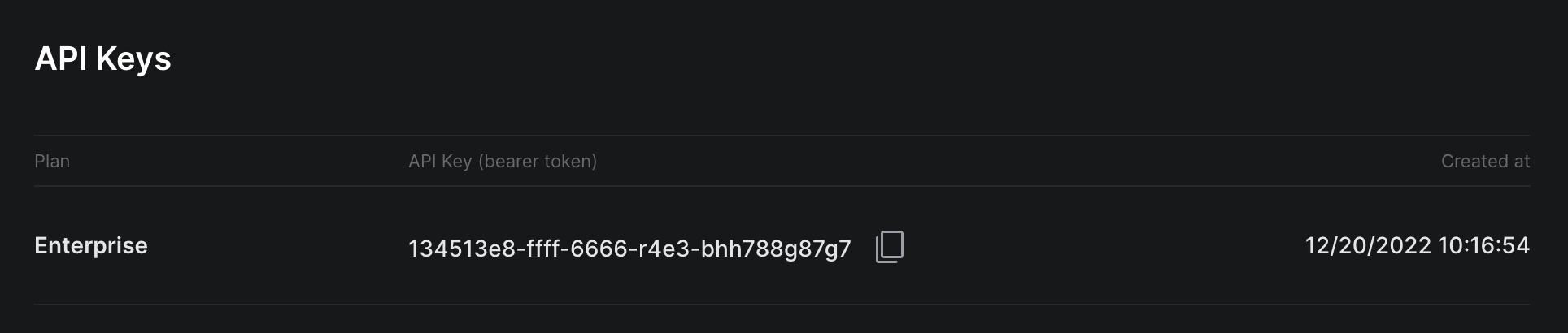
2. Install the SDK
npm i @hellomoon/api
yarn add @hellomoon/api
3. After installation - You can now import and use the SDK
const { TokenPriceStream } = require("@hellomoon/api");
Start Building Now - With Examples and Recipes
References
targetType
Hello Moon offers two targetType(s)
WEBSOCKET
WEBHOOK
They are both used to send real-time blockchain information, it is their communication protocol that separates them. For example, a websocket allows for a persistent connection between applications to exchange data. While a webhook, is a way for an application to provide other applications with occurred events. For example, you can setup an application that sends push alerts to a discord channel when wrapped solana's token price drops under 30 USDC.
Stream Classes
Stream Classes are used to create a new data stream. Checkout the links below for an explanation for each stream including its fields.
const stream = new TokenPriceStream({
target: {
targetType: "WEBSOCKET",
},
filters: {
mint: dataStreamFilters.text.equals(
"So11111111111111111111111111111111111111112"
),
},
name: "Alert when Wrapped Solana Drops Under 30 USDC",
})
dataStreamFilters
The dataStreamFilters object contains 6 types that is used to create your filters.
For example, dataStreamFilters.text is used since we only want to receive stream messages if the mint text equals So11111111111111111111111111111111111111112.
mint: dataStreamFilters.text.equals(
"So11111111111111111111111111111111111111112"
),
text
equals
notEquals
or
numeric
equals
notEquals
greaterThan
greaterThanEquals
lessThan
lessThanEquals
between
or
and
boolean
equals
date
equals
notEquals
or
is
and
boolean
composite
date
numeric
or
text
composite
type
filter
fields
Subscribe To A Websocket
1. Import StreamClient
Create a new StreamClient with your API_KEY
2. Get your API_KEY (bearer token)
Visit https://www.hellomoon.io/dashboard
3. Get your subscriptionId
Visit https://www.hellomoon.io/datastreams to copy and paste the subscriptionId
4. Start your stream!
Take your newly created StreamClient and call the function subscribe with the subscriptionId, to start receiving messages.
const { StreamClient } = require("@hellomoon/api");
const client = new StreamClient("REPLACE_WITH_HELLOMOON_API_KEY");
const main = async () => {
client
.connect((data) => {
// A fallback message catcher. This shouldn't fire, but can be used for system messages come through
console.log(data);
})
.then(
(disconnect) => {
const unsubscribe = client.subscribe(
"REPLACE_WITH_SUBSCRIPTION_ID",
(data) => {
// An array of streamed events
console.log(data);
}
);
},
(err) => {
// Handle error
console.log(err);
}
)
.catch(console.error);
};
main().then().catch();
Updated almost 2 years ago