Access a network of Solana Nodes, designed to build low latency, fast and inexpensive applications at scale. Retrieve Solana blockchain data, submit transactions, query account data and more with Hello Moon RPC today.
Our RPC endpoints:
- Mainnet beta: https://rpc.hellomoon.io
- Devnet: https://rpc-devnet.hellomoon.io
1 RPC call = 1 Credit
Table of Contents
Authentication
All you need to get your free Hello Moon API Key is a Solana wallet and browser extension for this product.
There are two ways to get your Hello Moon API Key
- Visit https://www.hellomoon.io/dashboard and connect your wallet to get your free API key.
For a detailed guide on getting your API Key visit: Get your API Key
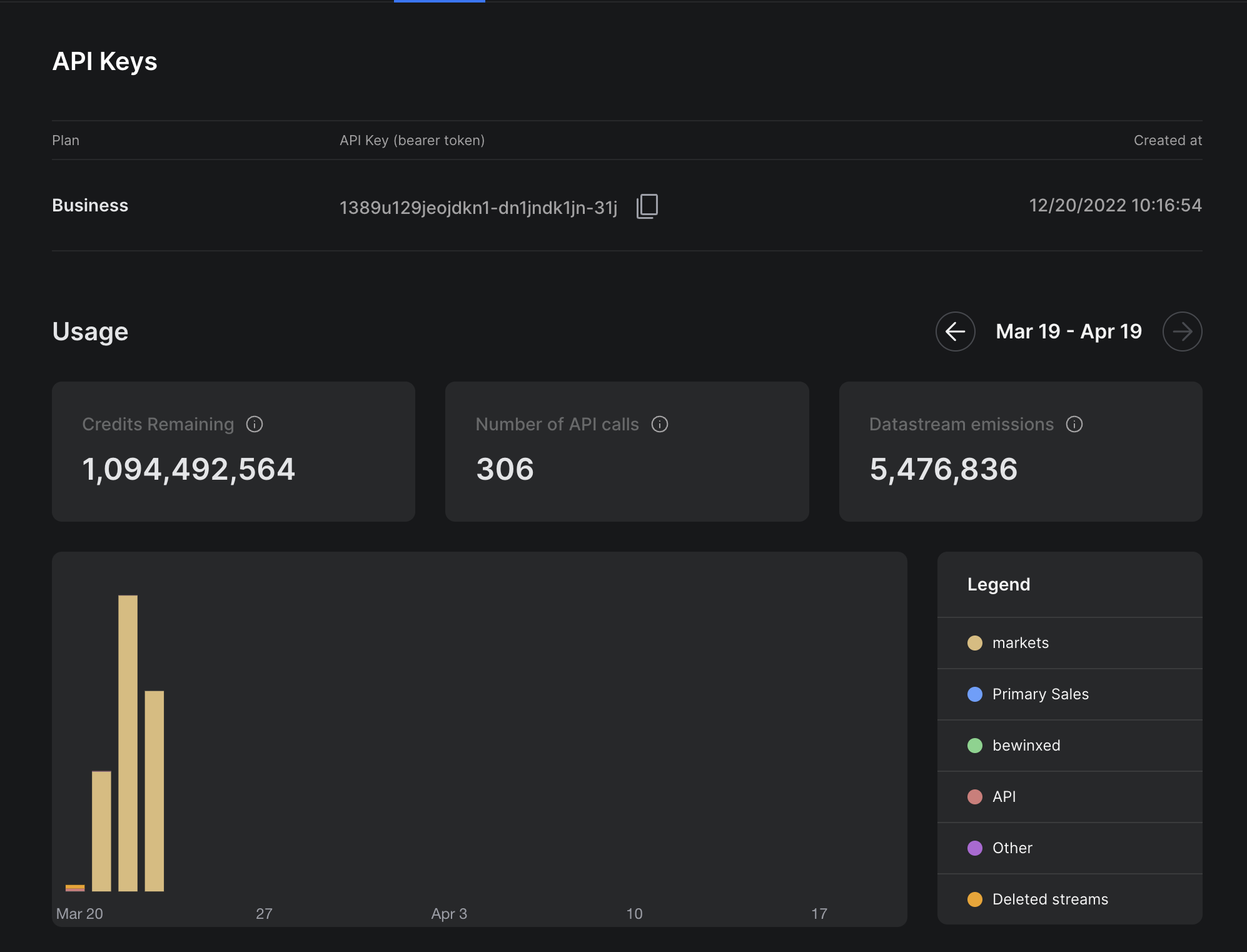
- Check the top right corner of readme (only visible for API request pages)
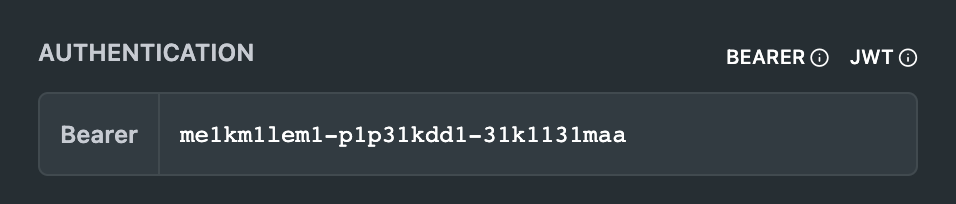
- To pass the API key to the RPC, add it to the end of the url like this: https://rpc.hellomoon.io/<API_KEY>
Building with Hello Moon SDK & Programming Languages
Build via your favorite programming language
require("dotenv").config();
const { Connection, PublicKey, LAMPORTS_PER_SOL } = require("@solana/web3.js");
const run = async () => {
const connection = new Connection(
`https://rpc.hellomoon.io/${process.env.HELLOMOON_API_KEY}`, // REPLACE process.env.HELLOMOON_API_KEY with your API key
);
const balance = await connection.getBalance(
new PublicKey("ANY_PUBLIC_KEY")
);
console.log(`Your Solana Balance is ${balance / LAMPORTS_PER_SOL} SOL`);
};
run().then().catch();
Success!, After a couple of seconds you should see a response.
{
"jsonrpc":"2.0",
"result": {
"context": {
"apiVersion": "1.14.13",
"slot": 203993889 // block id
},
"value": 999995000
},
"id":1
}
Test via Websocket and Command Line
- Install wscat - https://www.npmjs.com/package/wscat globally
npm install -g wscat
- Open a websocket connection with a persisted HELLOMOON_API_KEY
wscat -c wss://kiki-stream.hellomoon.io --auth user:REPLACE_HELLOMOON_API_KEY_HERE
- Once you see
Connected (press CTRL+C to quit)
you can enter the code below.
{
"jsonrpc": "2.0",
"id": 1,
"method": "getBalance",
"params": [
"8VrQeSRxymJBTsP6cd59dE5VGKECnsHo5nen3vCe631x"
]
}
- Success!, After a couple of seconds you should see a response.
{
"jsonrpc":"2.0",
"result": {
"context": {
"apiVersion": "1.14.13",
"slot": 203993889 // block id
},
"value": 999995000
},
"id":1
}
List of supported Solana RPC method calls
Some RPC method calls are supported, if you want a method to be added or other requests please visit https://discord.gg/hellomoon and checkout the #developer-support channel to request for help.
RPC Method Type | |
---|---|
HTTP Method | β |
Websocket Method | β |
Historical and Archived RPC data | β |